40 indexing using labels in dataframe
Pandas DataFrame Indexing: Set the Index of a Pandas Dataframe Python list as the index of the DataFrame In this method, we can set the index of the Pandas DataFrame object using the pd.Index (), range (), and set_index () function. First, we will create a Python sequence of numbers using the range () function then pass it to the pd.Index () function which returns the DataFrame index object. What does the pandas DataFrame.index attribute do? In pandas.DataFrame the row labels are called indexes, If you want to get index labels separately then we can use pandas.DataFrame "index" attribute. Example 1 In this example, we have applied the index attribute to the pandas DataFrame to get the row index labels.
How to get the names (titles or labels) of a pandas data frame in python To get the names of the data frame rows: >>> df.index Index(['Alice', 'Bob', 'Emma'], dtype='object') Get the row names of a pandas data frame (Exemple 2) Another example using the csv file train.csv (that can be downloaded on kaggle): >>> import pandas as pd >>> df = pd.read_csv('train.csv') >>> df.index RangeIndex(start=0, stop=1460, step=1)
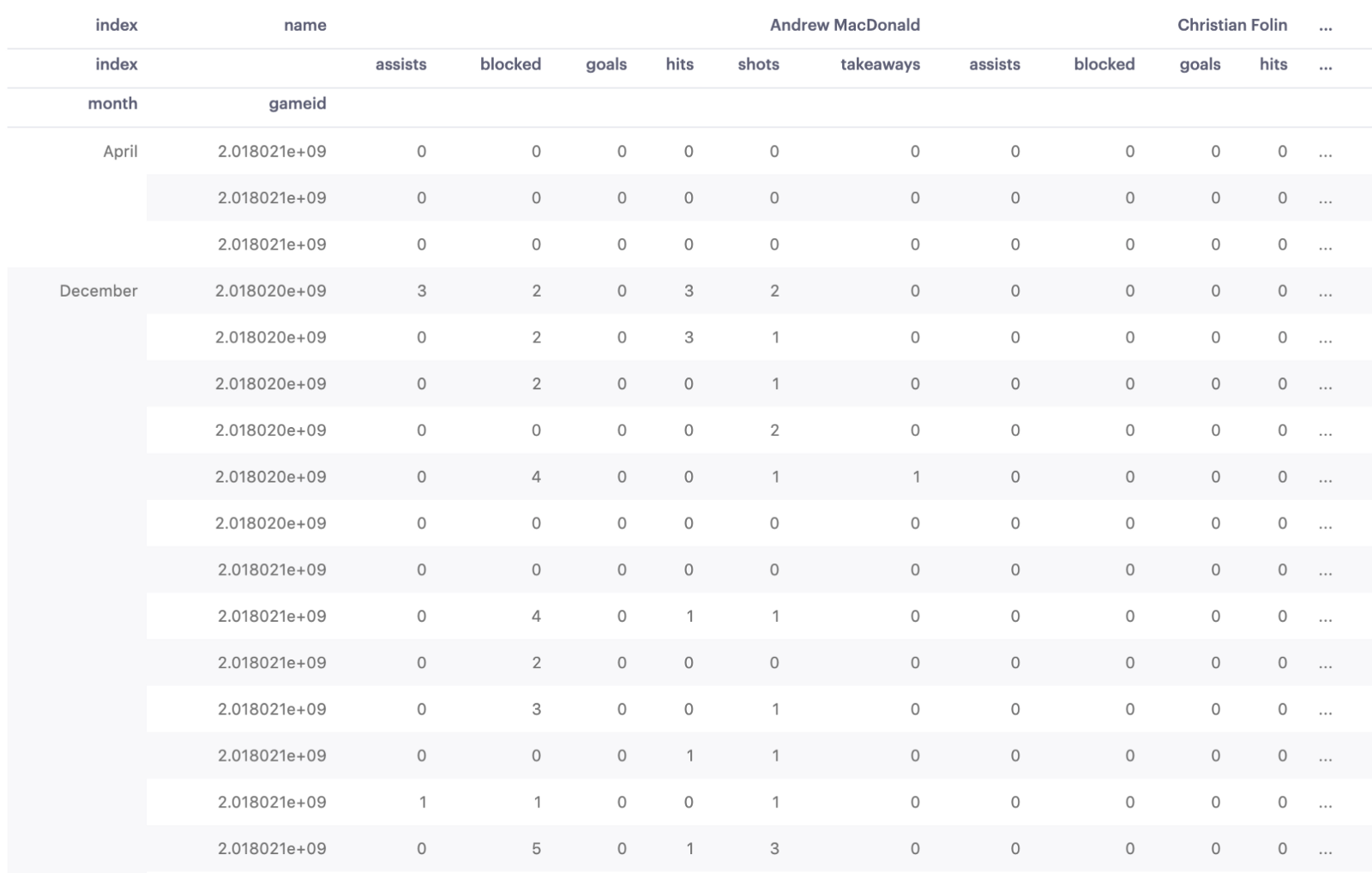
Indexing using labels in dataframe
How to Select Rows by Index in a Pandas DataFrame - Statology Often you may want to select the rows of a pandas DataFrame based on their index value. If you'd like to select rows based on integer indexing, you can use the .iloc function. If you'd like to select rows based on label indexing, you can use the .loc function. This tutorial provides an example of how to use each of these functions in practice. Pandas Index Explained with Examples - Spark by {Examples} Set Labels to Index The labels for the Index can be changed as shown in below. # Set new Index df. index = pd. Index (['idx1','idx2','idx3']) print( df. index) # Outputs # Index ( ['idx1', 'idx2', 'idx3'], dtype='object') 7. Get Rows by Index By using DataFrame.iloc [] property you can get the row by Index. Pandas DataFrame index and columns attributes - JournalDev Pandas DataFrame index and columns attributes allow us to get the rows and columns label values. We can pass the integer-based value, slices, or boolean arguments to get the label information. Pandas DataFrame index. Let's look into some examples of getting the labels of different rows in a DataFrame object.
Indexing using labels in dataframe. Pandas DataFrame Indexing - KDnuggets In pandas data frames, each row also has a name. By default, this label is just the row number. However, you can set one of your columns to be the index of your DataFrame, which means that its values will be used as row labels. We set the column 'name' as our index. It is a common operation to pick out one of the DataFrame's columns to work on. Indexing and Sorting a dataframe using iloc and loc Labels based indexing using loc. To index a dataframe based on column names, loc can be used. For example, to get all the columns between petal_length till iris class and records from 2nd to 10th, can be extracted by using - ... Simples way to sort a dataframe can be done using sort_values function of pandas dataframe, which take the column ... Indexing and selecting data — pandas 1.4.3 documentation pandas provides a suite of methods in order to have purely label based indexing. This is a strict inclusion based protocol. Every label asked for must be in the index, or a KeyError will be raised. When slicing, both the start bound AND the stop bound are included, if present in the index. Pandas: Create an index labels by using 64-bit integers, floating-point ... Have another way to solve this solution? Contribute your code (and comments) through Disqus. Previous: Write a Pandas program to display the default index and set a column as an Index in a given dataframe and then reset the index. Next: Write a Pandas program to create a DataFrame using intervals as an index.
Pandas : Sort a DataFrame based on column names or row index labels ... In the Python Pandas Library, the Dataframe section provides a member sort sort_index () to edit DataFrame based on label names next to the axis i.e. DataFrame.sort_index (axis=0, level=None, ascending=True, inplace=False, kind='quicksort', na_position='last', sort_remaining=True, by=None) Where, Pandas Indexing Examples: Accessing and Setting Values on DataFrames Some common ways to access rows in a pandas dataframe, includes label-based (loc) and position-based (iloc) accessing. ... loc example, string index. Use .loc[] to select rows based on their string labels: import pandas as pd # this dataframe uses a custom array as index df = pd. Working With Specific Values In Pandas DataFrame - Data Courses This function of a pandas DataFrame is of high value as you can build an index using a specific column, (meaning: a label) that you want to use for managing and querying your data. For example, one can develop an index from a column of values and then use the attribute.loc to select data from pandas DataFrame based on a value found in the index. Pandas Tutorials - loc() , set_index() , reset_index() - MLK - Machine ... This pandas function is used for setting the DataFrame index using existing columns. Syntax. pandas.DataFrame.set_index(keys, drop=True, append=False, inplace=False, verify_integrity=False) keys : label or array-like or list of labels/arrays - This parameter can be either a single column key, a single array of the same length as the calling ...
Tutorial: How to Index DataFrames in Pandas - Dataquest Let's explore four methods of label-based dataframe indexing: using the indexing operator [], attribute operator ., loc indexer, and at indexer. Using the Indexing Operator If we need to select all data from one or multiple columns of a pandas dataframe, we can simply use the indexing operator []. Indexing a Pandas DataFrame for people who don't like to remember things In pandas data frames, each row also has a name. By default, this label is just the row number. However, you can set one of your columns to be the index of your DataFrame, which means that its values will be used as row labels. We set the column 'name' as our index. It is a common operation to pick out one of the DataFrame's columns to work on. How to select subset of data with Index Labels in Python Pandas? With.iloc attribute,pandas select only by position and work similarly to Python lists. The .loc attribute selects only by index label, which is similarto how Python dictionaries work. Select a Subset Of Data Using Index Labels with .loc[] The loc and iloc attributes are available on both Series and DataFrame Set Index in pandas DataFrame - PYnative This function is used to re-assign a row label using the existing column of the DataFrame. It can assign one or multiple columns as a row index. Let's see how to use DataFrame.set_index() function to set row index or replace existing. Syntax. DataFrame.set_index(keys, drop=True, append=False, inplace=False, verify_integrity=False) Parameters
Indexing Dataframes. Indexing Dataframes in Pandas - Medium It is one of the most versatile methods in pandas used to index a dataframe and/or a series method.The loc () function is used to access a group of rows and columns by label (s) or a boolean array. loc [] is primarily label based, but may also be used with a boolean array. The syntax being:
How to Index Data in Pandas with Python - Medium Using Single Label. One way we can specify which rows and/or columns we want is by using labels. For rows, the label is the index value of that row, and for columns, the column name is the label. For example, in our ufo dataframe, if we want the fifth row only along with all the columns, we would use the following: ufo.loc [4, :]
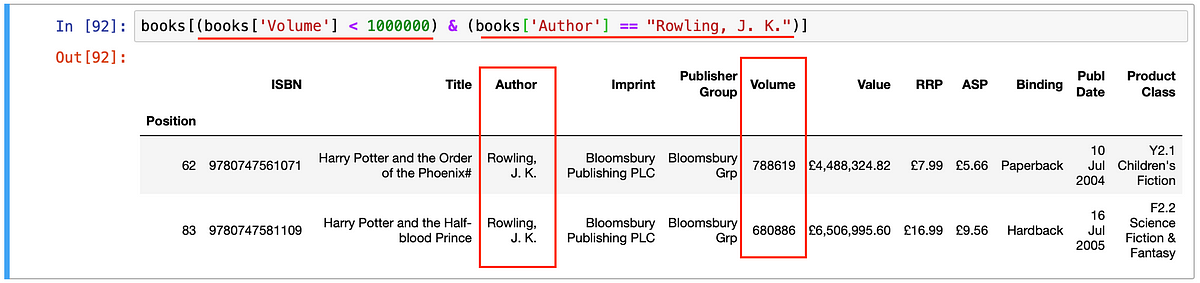
Filtering Data in Pandas. Using boolean indexing, filter, query… | by Mars Escobin | Level Up Coding
Indexing in Pandas Dataframe using Python - Medium Indexing using .loc method. If we use the .loc method, we have to pass the data using its Label name. Single Row To display a single row from the dataframe, we will mention the row's index name in the .loc method. The whole row information will display like this, Single Row information Multiple Rows
How to Select Columns by Index in a Pandas DataFrame If you'd like to select columns based on label indexing, you can use the .loc function. This tutorial provides an example of how to use each of these functions in practice. Example 1: Select Columns Based on Integer Indexing. The following code shows how to create a pandas DataFrame and use .iloc to select the column with an index integer ...

python - How to set_xticklabels to corresponding "dates" column of a pandas DataFrame? - Stack ...
pandas.DataFrame.set_index — pandas 1.4.3 documentation DataFrame.set_index(keys, drop=True, append=False, inplace=False, verify_integrity=False) [source] ¶ Set the DataFrame index using existing columns. Set the DataFrame index (row labels) using one or more existing columns or arrays (of the correct length). The index can replace the existing index or expand on it. Parameters
Indexing and Selecting Data with Pandas - GeeksforGeeks Indexing a DataFrame using .loc [ ] : This function selects data by the label of the rows and columns. The df.loc indexer selects data in a different way than just the indexing operator. It can select subsets of rows or columns. It can also simultaneously select subsets of rows and columns. Selecting a single row
Label-based indexing to the Pandas DataFrame - GeeksforGeeks In the above example, we use the concept of label based Fancy Indexing to access multiple elements of data frame at once and hence create two new columns ' Age ' and ' Marks ' using function dataframe.lookup () Example 3: Python3 import pandas as pd df = pd.DataFrame ( [ ['Date1', 1850, 1992,'Avi', 5, 41, 70, 'Avi'],
Python Pandas: Get Index Label for a Value in a DataFrame If I know the value in 'hair' is 'blonde', how do I get the index label (not integer location) corresponding to df.ix['mary','hair']? (In other words, I want to get 'mary' knowing that hair is 'blonde'). If I wanted the integer value of the index I'd use get_loc. But I want the label. Thanks in advance.
How to find index of value in Pandas dataframe - DevEnum.com 2. df.index.values to Find index of specific Value. To find the indexes of the specific value that match the given condition in Pandas dataframe we will use df ['Subject'] to match the given values and index. values to find an index of matched value. The result shows us that rows 0,1,2 have the value 'Math' in the Subject column.
Pandas Select Rows by Index (Position/Label) In this article, I will explain how to select rows from pandas DataFrame by integer index and label, by the range, and selecting first and last n rows with several examples. loc [] & iloc [] operators are also used to select columns from pandas DataFrame and refer to related article how to get cell value from pandas DataFrame.
Pandas DataFrame index and columns attributes - JournalDev Pandas DataFrame index and columns attributes allow us to get the rows and columns label values. We can pass the integer-based value, slices, or boolean arguments to get the label information. Pandas DataFrame index. Let's look into some examples of getting the labels of different rows in a DataFrame object.
Pandas Index Explained with Examples - Spark by {Examples} Set Labels to Index The labels for the Index can be changed as shown in below. # Set new Index df. index = pd. Index (['idx1','idx2','idx3']) print( df. index) # Outputs # Index ( ['idx1', 'idx2', 'idx3'], dtype='object') 7. Get Rows by Index By using DataFrame.iloc [] property you can get the row by Index.
How to Select Rows by Index in a Pandas DataFrame - Statology Often you may want to select the rows of a pandas DataFrame based on their index value. If you'd like to select rows based on integer indexing, you can use the .iloc function. If you'd like to select rows based on label indexing, you can use the .loc function. This tutorial provides an example of how to use each of these functions in practice.
Post a Comment for "40 indexing using labels in dataframe"